Building a Simple Web Application with Vue.js and Node.js: A Beginner’s Guide
Embarking on the journey of web development becomes both exciting and accessible with the combination of Vue.js and Node.js. Vue.js, a…
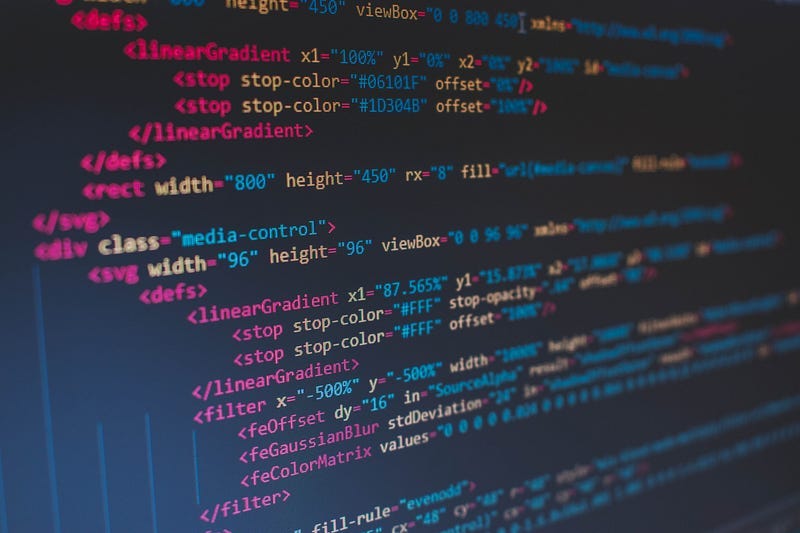
Embarking on the journey of web development becomes both exciting and accessible with the combination of Vue.js and Node.js. Vue.js, a progressive JavaScript framework, is renowned for its simplicity, while Node.js provides a robust server-side runtime. In this tutorial, we’ll guide you through the steps to build a straightforward web application using these technologies.
Prerequisites:
Before we begin, ensure you have the following installed on your system:
Node.js (https://nodejs.org/)
npm (Node Package Manager, comes with Node.js)
Step 1: Setting Up the Project
Open your terminal and create a new directory for your project. Navigate into the project directory:
bashCopy code
mkdir mywebapp
cd mywebapp
Step 2: Initializing Node.js
Initialize a new Node.js project using:
bashCopy code
npm init -y
This command creates a package.json
file with default settings.
Step 3: Installing Express
Install Express, a web application framework for Node.js, using the following command:
bashCopy code
npm install express
Step 4: Creating the Server
Create a file named server.js
in your project directory. Open it and write a simple server using Express:
javascriptCopy code
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello from Node.js!');
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
Step 5: Running the Server
Run your server with:
bashCopy code
node server.js
Visit http://localhost:3000
in your web browser to see your "Hello from Node.js!" message.
Step 6: Installing Vue.js
Now, let’s integrate Vue.js into our project. Install Vue.js using:
bashCopy code
npm install vue
Step 7: Creating Vue Components
Create a new directory named public
in your project. Inside public
, create an HTML file, for example, index.html
:
htmlCopy code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Vue.js App</title>
</head>
<body>
<div id="app">
<h1>{{ message }}</h1>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue@2"></script>
<script src="main.js"></script>
</body>
</html>
Inside the public
directory, create a JavaScript file named main.js
:
javascriptCopy code
new Vue({
el: '#app',
data: {
message: 'Hello from Vue.js!',
},
});
Step 8: Connecting Vue.js to the Server
Update your server.js
to serve the index.html
file:
javascriptCopy code
const express = require('express');
const app = express();
const port = 3000;
app.use(express.static('public'));
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
Step 9: Running the Application
Restart your server with:
bashCopy code
node server.js
Visit http://localhost:3000
again, and you'll see your Vue.js message in action!
Conclusion:
You’ve successfully built a simple web application using Vue.js and Node.js. This basic example lays the foundation for more complex projects, where you can explore Vue.js components, routing, and state management. The combination of Vue.js on the client-side and Node.js on the server-side offers a seamless development experience. Happy coding!